Select pizza
This is a part of the tutorial on creating a button-based bot that can order pizza.
- Selecting a delivery city
- Creating a dictionary
- Selecting a pizza (you are here)
- Making an order
- Testing the bot script
At the previous step, we’ve created the pizza.csv
dictionary file that lists available types of pizza in the delivery cities.
Now, we’ll be creating a script for the client to select a pizza and make an order.
Creating a script
Create a pizza.sc
script file in the src
folder and paste the following:
require: pizza.csv
name = pizza
var = pizza
theme: /
state: ChoosePizza
a: What pizza would you like to order?
script:
for (var id = 1; id < Object.keys(pizza).length + 1; id++) {
var regions = pizza[id].value.region;
if (_.contains(regions, $client.city)) {
var button_name = pizza[id].value.title;
$reactions.buttons({text: button_name, transition: 'GetName'})
}
}
state: GetName
script:
$session.pizza_name = $request.query;
go!: /ChooseVariant
state: ClickButtons
q: *
a: Please, click the button.
go!: ..
state: ChooseVariant
a: Please, select an option:
script:
for (var id = 1; id < Object.keys(pizza).length + 1; id++) {
if ($session.pizza_name == pizza[id].value.title) {
var variations = pizza[id].value.variations;
for(var i = 0; i < variations.length; i++){
var button_name = variations[i].name + " for " + variations[i].price + " $"
$reactions.inlineButtons({text: button_name, callback_data: variations[i].id })
}
}
}
a: Click "Menu" to return to pizza selection
buttons:
"Menu" -> /ChoosePizza
state: ClickButtons
q: *
a: Please, click the button.
go!: ..
state: GetVariant
event: telegramCallbackQuery
script:
$session.pizza_id = parseInt($request.query);
go!: /ChooseQuantity
state: ChooseQuantity
a: Please select the number of pizzas:
buttons:
"1" -> ./GetQuantity
"2" -> ./GetQuantity
"3" -> ./GetQuantity
state: ClickButtons
q: *
a: Please, click the button.
go!: ..
state: GetQuantity
script:
$session.quantity = parseInt($request.query);
$session.cart.push({name: $session.pizza_name, id: $session.pizza_id, quantity: $session.quantity});
a: Do you want to choose something else, or proceed to checkout?
buttons:
"Menu" -> /ChoosePizza
buttons:
"Proceed to checkout" -> /Cart
state: ClickButtons
q: *
a: Please, click the button.
go!: ..
Adding modules
At the beginning of the script, include the pizza.csv
dictionary under the require
tag.
Here, var = pizza
indicates that the dictionary is accessible further via the pizza
keyword
require: pizza.csv
name = pizza
var = pizza
Now, let’s connect the pizza.sc
script to the main.sc
script:
require: pizza.sc
States
The script consists of a few states:
ChoosePizza
— selecting pizza from thepizza.csv
dictionary.GetName
— saving the client’s option.ChooseVariant
— selecting a pizza variant.GetVariant
— saving parameters for the selected pizza.ChooseQuantity
— selecting the number of pizzas to order.GetQuantity
— saving the entered number of pizzas.
Script structure
ChoosePizza
At this step, the client has already selected the city where they want to order pizza. So, we display a list of pizzas available in the selected city.
state: ChoosePizza
a: What pizza would you like to order?
script:
# displaying pizza variations in the selected city
for (var id = 1; id < Object.keys(pizza).length + 1; id++) {
var regions = pizza[id].value.region;
if (_.contains(regions, $client.city)) {
var button_name = pizza[id].value.title;
$reactions.buttons({text: button_name, transition: 'GetName'})
}
}
To display available pizza variants, refer to the pizza.csv
dictionary and select the names of pizzas that can be ordered in the selected city.
Next, add the buttons that will perform the transition to the GetName
state using the $reactions.buttons
method.
GetName
Now, let’s save the client’s choice. In the GetName
state, assign the name of the selected pizza to the $session.pizza_name
variable. We can use it to access information about the pizza and display it in the cart. Then perform a transition to the ChooseVariant
state.
state: GetName
script:
$session.pizza_name = $request.query;
go!: /ChooseVariant
ChooseVariant
In the script
of the ChooseVariant
state, we create buttons to select pizza type. This time we use the $reactions.inlineButtons
method. Unlike $reactions.buttons
, these buttons are displayed inside the dialog as bot responses.
state: ChooseVariant
a: Please, select an option:
script:
for (var id = 1; id < Object.keys(pizza).length + 1; id++) {
if ($session.pizza_name == pizza[id].value.title) {
var variations = pizza[id].value.variations;
for(var i = 0; i < variations.length; i++){
var button_name = variations[i].name + " for " + variations[i].price + " $"
$reactions.inlineButtons({text: button_name, callback_data: variations[i].id })
}
}
}
a: Click "Menu" to return to pizza selection
buttons:
"Menu" -> /ChoosePizza
GetVariant
In the GetVariant
state, the telegramCallbackQuery
event is triggered by a click on the inline button from the ChooseVariant
state. In the script, assign the pizza parameters to the $session.pizza_id
variable. We can use it to access information about the pizza and display it later in the cart. Next, we move to the ChooseQuantity
state to select the number of pizzas.
state: GetVariant
event: telegramCallbackQuery
script:
$session.pizza_id = parseInt($request.query);
go!: /ChooseQuantity
ChooseQuantity
In the ChooseQuantity
state, the client selects the number of pizzas by clicking on the corresponding button. The dialog switches to the GetQuantity
state.
state: ChooseQuantity
a: Please select the number of pizzas:
buttons:
"1" -> ./GetQuantity
"2" -> ./GetQuantity
"3" -> ./GetQuantity
GetQuantity
In the script for the GetQuantity
state, assign the number of the selected pizzas to the $session.quantity
variable, and also add the selected option to the cart using the $session.cart.push
method.
Next, add two buttons, Menu and Checkout. A client can go back to the menu or to the cart to process the order.
state: GetQuantity
script:
$session.quantity = parseInt($request.query);
$session.cart.push({name: $session.pizza_name, id: $session.pizza_id, quantity: $session.quantity});
a: Do you want to choose something else, or proceed to checkout?
buttons:
"Menu" -> /ChoosePizza
buttons:
"Proceed to checkout" -> /Cart
ClickButtons
Let’s add a nested ClickButtons
state to ChoosePizza
, ChooseVariant
and ChooseQuantity
states. It performs the same way as in the main.sc
script.
state: ClickButtons
q: *
a: Please, click the button.
go!: ..
Testing
Let’s test the result in the Telegram.
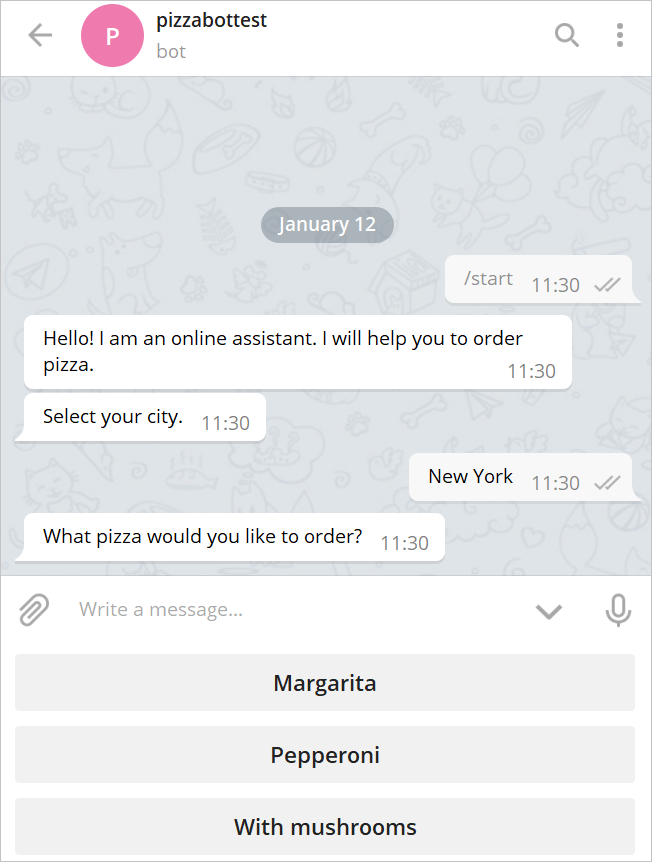
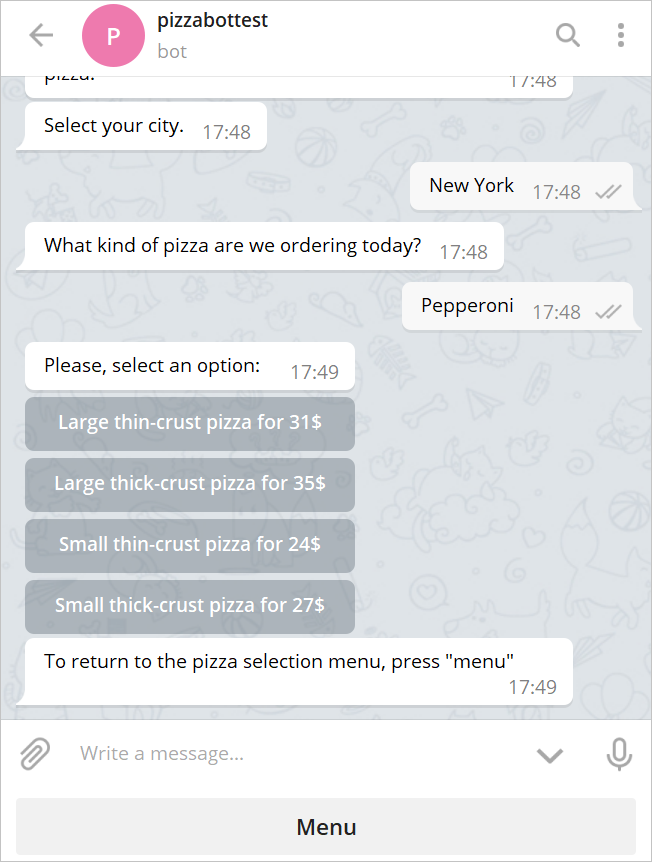
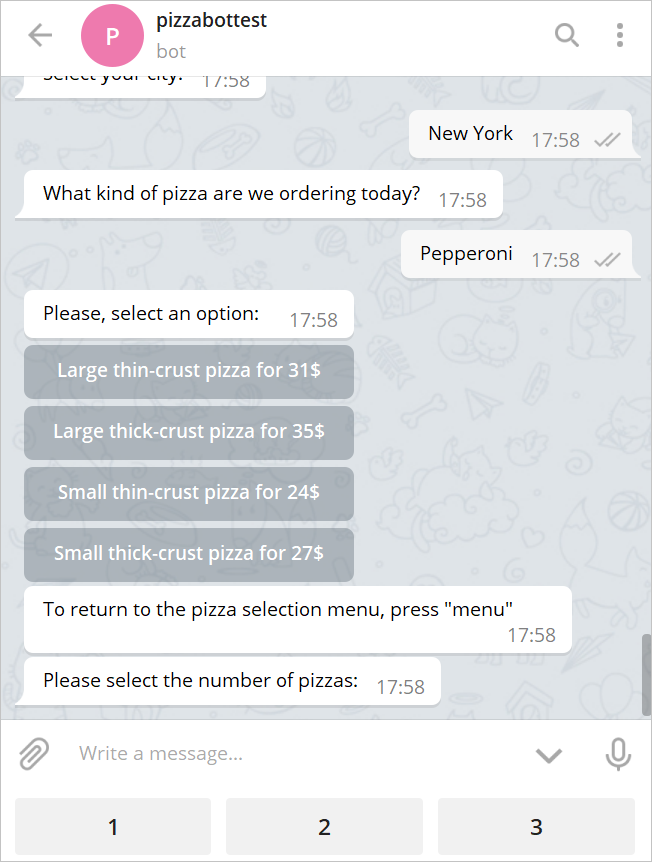
Next, move on to making an order.