Select a delivery city
This is a part of the tutorial on creating a button-based bot that can order pizza.
- Selecting a delivery city (you are here)
- Creating a dictionary
- Selecting a pizza
- Making an order
- Testing the bot script
At the first step, we will create a configuration file and a main script. The bot will offer a client to select the delivery city using two buttons, New York and Washington.
Configuration file
Create a chatbot.yaml
bot configuration file and set the following parameters:
# Project name
name: pizza-bot
# Main file
entryPoint:
- main.sc
# NLU parameters:
botEngine: v2 # Bot engine version
language: en # Bot language
nlp:
intentNoMatchThresholds:
phrases: 0.2
patterns: 0.2
Creating a script
Create a main.sc
file in the src
folder. It will contain the main bot script.
theme: /
state: Start
q!: $regex</start>
script:
$context.session = {}
$context.client = {}
$context.temp = {}
$context.response = {}
a: Hello! I am an online assistant. I will help you to order pizza.
go!: /ChooseCity
state: ChooseCity || modal = true
a: Select your city.
buttons:
"New York" -> ./RememberCity
"Washington" -> ./RememberCity
state: RememberCity
script:
$client.city = $request.query;
$session.cart = [];
go!: /ChoosePizza
state: ClickButtons
q: *
a: Please, click the button.
go!: ..
state: CatchAll || noContext=true
event!: noMatch
a: I don’t understand you.
The script consists of a few main states:
Start
— start of the script. The bot welcomes the client and offers them to select a delivery city.ChooseCity
— selecting a city from the two proposed options.RememberCity
— remembering the selected city.ClickButtons
— notifying the client that the bot understands only button clicks.CatchAll
— the state is used when the client’s message doesn’t fit any of the described stages.
Script structure
Start
The script starts with the Start
state. The bot sends a welcome message and offers the client to order pizza.
state: Start
q!: $regex</start>
script:
$context.session = {}
$context.client = {}
$context.temp = {}
$context.response = {}
a: Hello! I am an online assistant. I will help you to order pizza.
go!: /ChooseCity
The bot must forget the client’s answers from the previous dialog, so the script
here resets variables to start a new one. The previous answers are stored in the $context
object.
The transition to the ChooseCity
state is performed using the go!
tag.
ChooseCity
state: ChooseCity || modal = true
a: Select your city.
buttons:
"New York" -> ./RememberCity
"Washington" -> ./RememberCity
A client selects one of the cities using the New York and Washington buttons. To create a button use the buttons
tag.
The nested data of the buttons
tag matches the <json-node> -> <string>
pattern. <json-node>
is a string or object that defines a button text. <string>
is a line defining a transition path when the button is clicked. In the script, the transition is made to the RememberCity
state.
The modal=true
is used to prevent the bot from moving to the next state until the client selects a city.
RememberCity
state: RememberCity
script:
$client.city = $request.query;
$session.cart = [];
go!: /ChoosePizza
The client’s selection is processed with the $request.query
method and is written to the $client.city
variable. The bot offers the client further options depending on the selected city.
The transition to the ChoosePizza
state is performed using the go!
tag. We will take a look at the ChoosePizza
state in the following steps.
ClickButtons
Since we have set the modal
flag to true
in the ChooseCity
state, we need to create a new state reacting to any client’s message that differs from all the considered options in the ChooseCity
state.
In case the client writes a message instead of clicking the button to select a city, the bot displays: Please, click the button
.
state: ClickButtons
q: *
a: Please, click the button.
go!: ..
Since the information about the delivery city is still required for the conversation to go on, the bot returns to the ChooseCity
state using the go!
tag. ..
indicates that the transition is made to a parent state.
CatchAll
state: CatchAll || noContext=true
event!: noMatch
a: I don’t understand you.
People can make mistakes when typing commands and send the bot a text that differs from all considered options. For this purpose, the CatchAll
state is used, which processes the end of the script in case the client’s message doesn’t fit any of the described stages.
To prevent changing the context when a client gets into the CatchAll
state, set the noContext
flag to true
.
Testing
Let’s test the result in the Telegram channel.
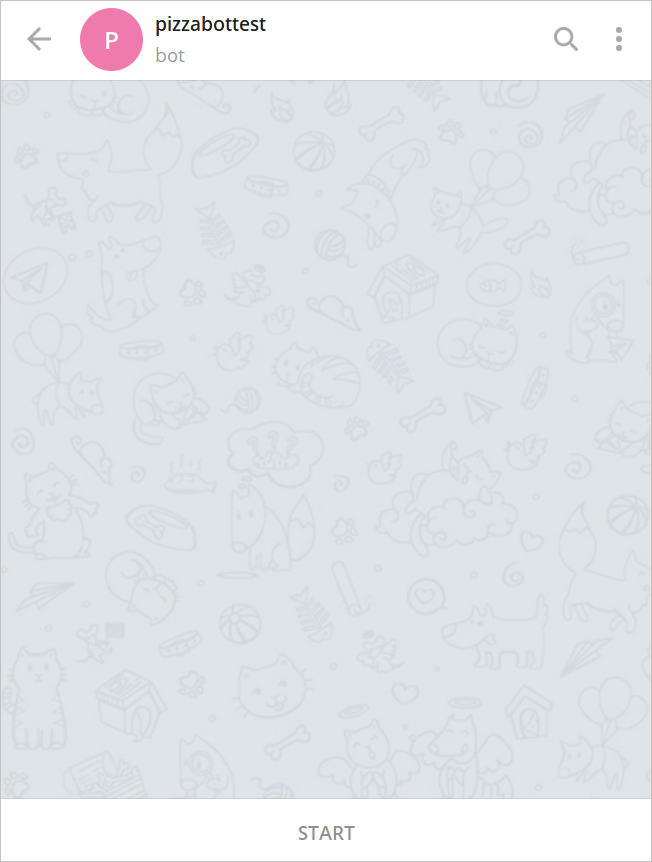
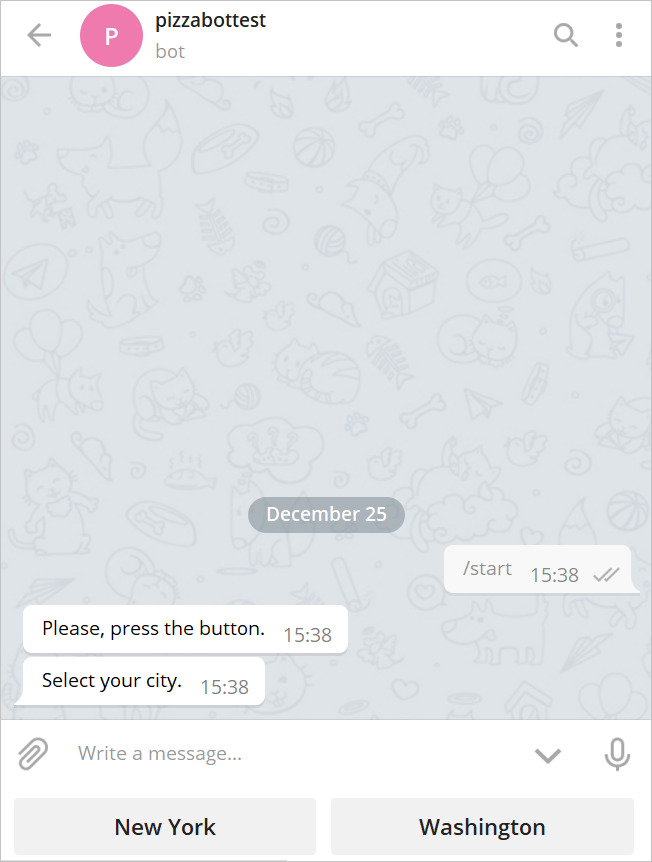
Next, move on to working with a pizza dictionary.