Conditions block
The Conditions block directs your bot to a certain screen depending on the condition fulfilled.
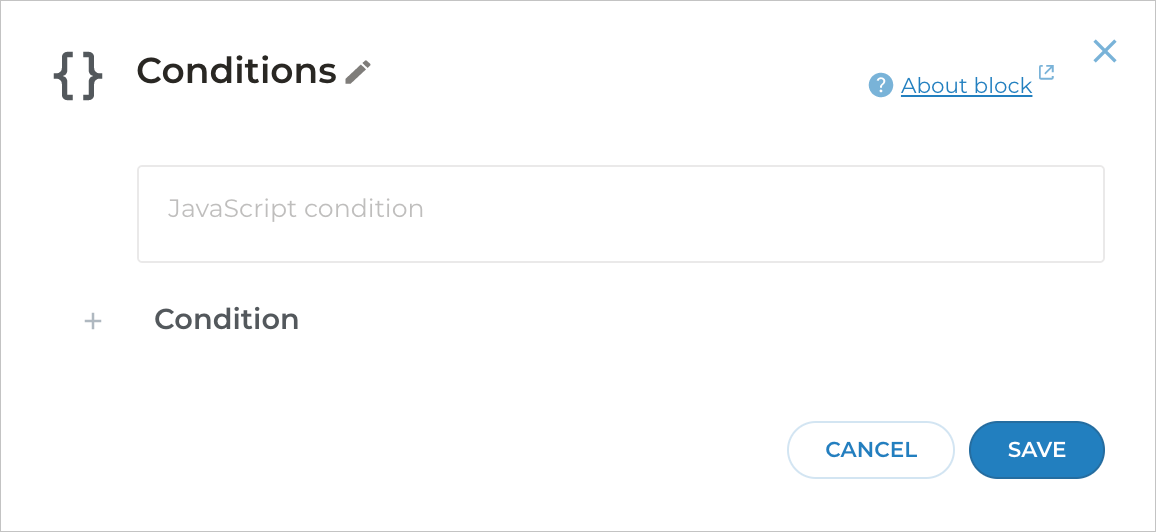
Parameters
You can use any JavaScript expressions in your Conditions block. You can specify any number of conditions with the transition to the desired screen for each of them. This way you can build non-linear dialog logic.
Adding multiple conditions
If you add multiple conditions one under the other to a single Conditions block, these conditions will be evaluated from top to bottom.
If the first (topmost) condition is fulfilled, the bot will follow the branch of this condition and ignore other conditions under it.
If the topmost condition is not fulfilled, the bot will proceed to evaluate the second one. This will continue until one of the conditions is fulfilled. As soon as any of the conditions is fulfilled, all the expressions below it will be ignored.
Each condition has a separate exit from the block which can be connected to different screens. Therefore, if each expression in the Conditions block is connected to the separate screen, the number of branches from the block will be equal to the number of the conditions, plus the else
condition.
Else
You will see a special else
condition in the Conditions block you add to your screen. This branch is followed if none of the conditions in your block is true.
Variables
You can use variables received by the bot in the previous screens or any Aimylogic system variables.
JavaScript
You can use any JavaScript operators and functions in your conditions. You can also use the functions from the Underscore library in complex situations where you need various data conversions (for objects, arrays, etc.).
Examples
Assignment
Use the =
operator to assign a value to a variable.
If you want to assign some text to a variable, enclose the text in quotation marks, e.g., $choice = "buy"
.
This condition will assign the value of buy
to the $choice
variable.
You do not need to use quotation marks with numbers you assign to variables, e.g., $age = 20
.
Assigning zero
When you assign 0
to the variable, the expression is written in the same way as when you assign any other number:
$score = 0
The a = 1
expression is interpreted as true
in the JavaScript specification, while a = 0
is interpreted as false
. Therefore, if you assign 0
, the bot will follow the else
branch.
You need to associate both true
and else
with the same screen if:
- you assign
0
to the variable; - you assign another variable to your variable, and it may be
0
; - you assign the number with the integer part equal to
0
, e.g.,$score = 0.08
; - you put the text or the number entered by the client into the variable, and you suppose the client may enter
0
.
This is due to the JavaScript specifics and is necessary for assigning 0
correctly.
Arithmetic operations
You can perform arithmetic operations in your Conditions block. In JavaScript, you can sum not only numbers but strings (text) as well. For example, ab
will be output here in the result of string variables addition:
Comparison
You can use the ===
operator for comparison, e.g., $score === 5
will be true if $score
equals five.
The ===
operator also checks the data type — unlike the ==
operator that casts the values to the same type.
You can use the ||
or &&
operators to evaluate two conditions at once.
One of the conditions is true, e.g., $choice === "yes" || $choice === "maybe"
.
This condition will be true if $choice
is equal to either “yes” or “maybe”.
Clicking a button is equal to text input, so after the user clicks “yes” the value of “yes” will be saved to the $choice
variable and the condition will be fulfilled.
Multiple operations on one line
You can combine several operations on one line with the help of ||
or &&
operators.
Checking multiple conditions
One condition is true
||
shows that one condition from all is true. For example:
$choice === "yes" || $choice === "maybe"
Such condition will work if $choice
is either “yes” or “maybe”.
So as pressing the button is equivalent to text input, after pressing the “yes” button the “yes” value will be placed in the$choice
variable. After that the condition will be executed.
Both conditions are true
&&
shows that both conditions are true. For example:
$kids >= 2 && $kids <= 5
The condition will be true if $kids
equals 2, 3, 4, or 5. I.e., $kids
must be not less than 2 or greater than 5.
Assigning multiple variables
To declare multiple variables on one line, you should enclose each operation in brackets and put the &&
operator between operations:
($color1 = "blue") && ($color2 = "red") && ($color3 = "green")
As the result of condition execution, the bot will declare three variables: $color1
, $color2
, and $color3
.
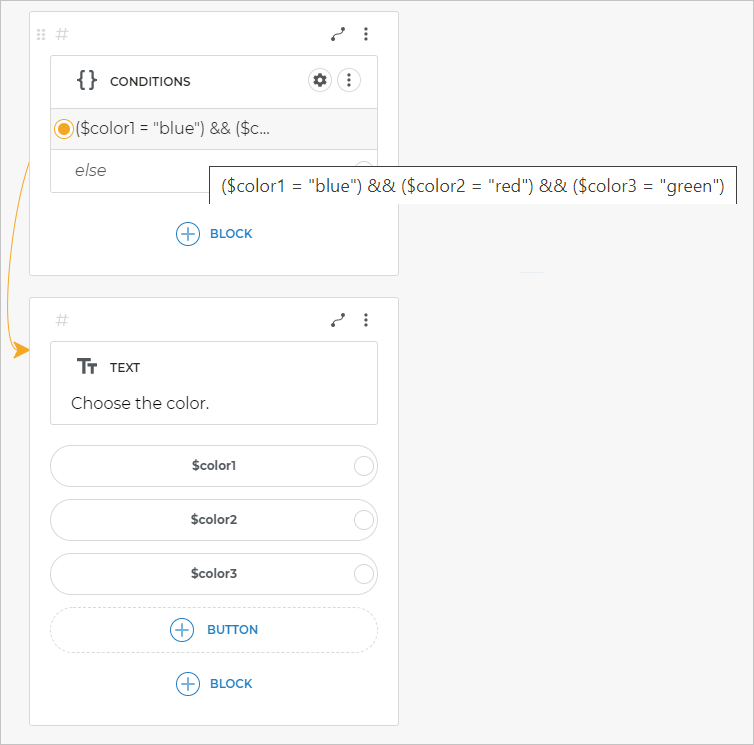
Performing multiple arithmetic operations
To perform multiple arithmetic operations, you should enclose each operation in brackets and put the &&
operator between operations.
Let’s assume that we have assigned numerical values to $num1
and $num2
variables. To get both sum and difference of these numbers using one line condition, let’s perform these operations in new variables using the following expression:
($num3 = $num1 + $num2) && ($num4 = $num1 - $num2)
As the result of condition execution, the bot will put the sum of $num1
and $num2
variables into the $num3
variable, and also put the difference of these variables into the $num4
variable.
JavaScript conditional operator
The conditional (ternary) JavaScript operator is the only JavaScript operator that uses three operands. The operator takes one of the two values depending on the condition specified. The operator syntax:
condition ? val1 : val2
For example:
$name = $NAME ? $NAME.name : $isname
We use the =
operator to assign a value to the $name
variable in this condition.
If we have the $NAME
variable, the value of $NAME.name
will be written to $name
.
Otherwise, the value of $isname
will be written to $name
.
You can also use the conditional operator in the following way:
-
Request client’s age with the help of the
$num to var
block, assign it to the$age
variable. -
Specify the following condition:
$status = ($age >= 18) ? "adult" : "child"
.
We use the =
operator to assign a value to the $status
variable here.
We evaluate the $age >= 18
condition, i.e. the number stored in $age
must be equal to or greater than 18. If the number stored in $age
is equal to or greater than 18, it evaluates to true
and the first condition is fulfilled: the value of adult
will be written to $status
.
If the number stored in $age
is less than 18, “child” will be written to $status
.
Using JavaScript methods
You can also use various JavaScript methods to perform arithmetic operations in the Conditions block.
For example, the .toFixed
method rounds the number to the desired decimals.
$roundup = $num.toFixed(2)
In this case, the .toFixed
method will round the number contained in the $num
variable to the second decimal. The resulting value will be written to the $roundup
variable.
For the case the integer part of the number is zero, the assignment should be made by associating both true
and else
with the desired screen.