HTTP request block
You can use the HTTP request block to exchange data with external resources and save it into variables.
Parameters
To add the block to the script, select HTTP request from the block menu.
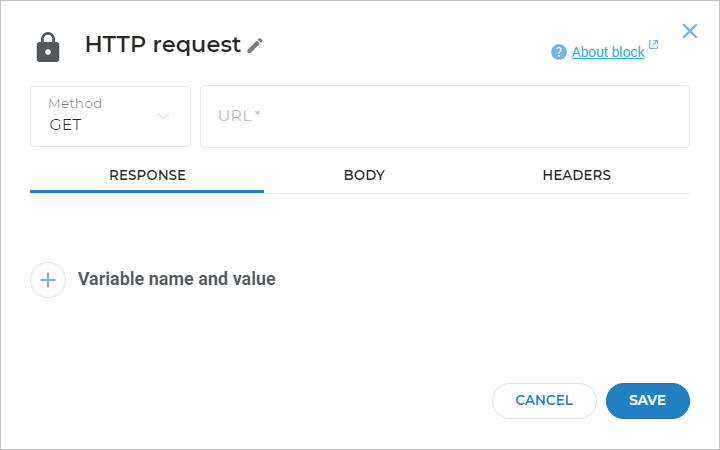
Configure the required parameters:
- Select the method for your request:
GET
(by default)POST
DELETE
PUT
- Specify the request URL.
Also, you can fill out the RESPONSE, BODY, and HEADERS tabs.
URL
The bot will use the specified URL to send and receive data.
You can add variables to a URL in the following ways:
https://example.com?query=${varname}
https://${varname}/endpoint
${varname}
(if you store the whole URL in the variable)
Here, varname
is a variable name.
%7B
and %7D
for the left and the right brace respectively.To add the text of the last client’s input to the URL, specify queryText
as a variable name, for example: https://example.com?query=${queryText}
.
The bot will automatically insert its value into the URL before executing the request.
$queryText
is the system variable that stores the current user request.In such a way, for example, you can read data from different Google Sheets without creating several HTTP requests.
Headers
On the HEADERS tab, you can add request headers by filling out the Header and Value fields.
You can also use variables in headers.
$
in front of variable names.Request body
For all request methods, you can fill out the request body on the BODY tab.
In the request body, you can specify data in any format (JSON, XML, plain text) and use variables.
In the example above, the request body is a JSON object.
The bot will automatically insert the $age
and $name
values into the request body.
Here, the
$age
variable is specified without quotation marks because it contains a number. The $name
variable is specified with quotation marks because it contains a string.Response
In the response, the server usually returns data that you can parse or send to clients.
On the RESPONSE tab, you can define which data from the response should be saved to variables.
For example, a request to the https://api.forismatic.com/api/1.0/?method=getQuote&format=json&lang=en
URL returns the following JSON object:
{
"quoteText": "Text",
"quoteAuthor": "Author",
"senderName": "Publisher",
"senderLink": "Profile link",
"quoteLink": "Link to the quote at the website"
}
This JSON object is stored in the $httpResponse
system variable.
To save data from a particular field from the response, specify:
- The variable name you want to save data to.
- The variable value via the
$httpResponse
system variable.
If the request succeeds, the bot will create the $quoteText
and $quoteAuthor
variables with the server response fields as values.
httpResponse
Aimylogic saves the server response to the $httpResponse
system variable.
If the server response is in the JSON or XML format, Aimylogic will automatically convert it to a JavaScript object that you can process with JavaScript functions.
For example, to save the quoteText
value to a new variable, you need to specify $httpResponse.quoteText
as the variable value.
Here, quoteText
is the name of the field you need to get.
If the server returns a non-JSON and non-XML string, this entire string will be stored in $httpResponse
without any processing.
JavaScript
When creating variables, you can use JavaScript expressions and functions, as well as functions from the Underscore library, which makes it easier to work with objects and arrays.
For example, the server returns the following JSON object as a response:
{
"items": [
{
"name": "oranges",
"price": 150
},
{
"name": "tangerines",
"price": 200
}
]
}
Also, let’s suppose the bot has asked the client which fruit they need and stored it in the $choice
variable.
To save the object from items
to a variable, you will need the following JavaScript expression:
_.findWhere($httpResponse.items, {name: "$choice"})
The function will look for the item that matches the $choice
value by the name
field.
Transitions from the block
The HTTP request block has two transitions:
- Success if the server returns a response with the code in range from
200
to299
inclusive. - Error if the server returns any other response.
$httpStatus
system variable.